Speed Up Your Website with Lighter Images
Make your website faster with simplest image optimization tool to improve web performance and save time. It takes one click to resize, compress and convert your images to WebP.
Make your website faster
Images can account for 50% of your loading time.
By compressing them, you will gain precious seconds
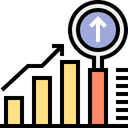
Enhance user experience
A fast web page encourages your visitors to stay on your website and keep browsing. Offer them an ultrafast experience!
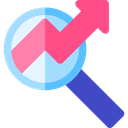
SEO performance
A fast website also means increasing the SEO visibility on Google – which can bring more high-quality traffic.
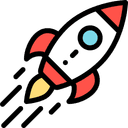
Boost Conversions
With more visitors and more engagement, you will naturally have more subscribers and more sales!
Developer API
Automate your WebP, JPEG and PNG compression workflow
Same API for WebP, JPEG & PNG images
The API compresses WebP, JPEG and PNG images. You only have to upload your source image and download the result. Everything else happens automatically.
API Details
https://api.image.antuan01.com/convert
METHOD: POST/form-data
FIELDS: file => "the image file"
Code Examples
import requests
url = 'https://api.image.antuan01.com/convert'
image = {'file': open('image.png' ,'rb')}
res = requests.post(url, files = image)
handle = open('image.webp', 'wb')
handle.write(res.content)
handle.close()
package main
import (
"bytes"
"io"
"log"
"mime/multipart"
"net/http"
"os"
"time"
)
func main() {
client := &http.Client{
Timeout: time.Second * 10,
}
body := &bytes.Buffer{}
writer := multipart.NewWriter(body)
fw, err := writer.CreateFormFile("file", "chiguire2.png")
if err != nil {
log.Printf("Error %d", err.Error)
}
file, err := os.Open("chiguire2.png")
if err != nil {
log.Printf("Error %d", err.Error)
}
_, err = io.Copy(fw, file)
if err != nil {
log.Printf("Error %d", err.Error)
}
writer.Close()
req, err := http.NewRequest("POST", "https://api.image.antuan01.com/convert", bytes.NewReader(body.Bytes()))
if err != nil {
log.Printf("Error %d", err.Error)
}
req.Header.Set("Content-Type", writer.FormDataContentType())
rsp, _ := client.Do(req)
if rsp.StatusCode != http.StatusOK {
log.Printf("Request failed with response code: %d", rsp.StatusCode)
}
tmpfile, err := os.Create("./imageGo.webp")
defer tmpfile.Close()
if err != nil {
log.Printf("Err: %d", err)
}
_, err = io.Copy(tmpfile, rsp.Body)
if err != nil {
log.Printf("Err: %d", err)
}
}
require "net/http"
require "uri"
uri = URI('https://api.image.antuan01.com/convert')
request = Net::HTTP::Post.new(uri)
form_data = [['file', File.open("./image.png")]]
request.set_form form_data, 'multipart/form-data'
response = Net::HTTP.start(uri.hostname, uri.port, use_ssl: true) do |http|
http.request(request)
end
File.write("image.webp", response.body, mode: "wb")
require 'vendor/autoload.php';
use GuzzleHttpPsr7;
use GuzzleHttpClient;
$client = new Client();
$response = $client->request('POST', 'https://api.image.antuan01.com/convert', [
'multipart' => [
[
'name' => 'file',
'contents' => Psr7Utils::tryFopen('image.png', 'r')
],
]
]);
$myfile = fopen("image.webp", "wb") or die("Unable to open file!");
fwrite($myfile, $response->getBody());
fclose($myfile);
const fs = require('fs');
const path = require('path')
const fetch = require('node-fetch');
const FormData = require('form-data');
const formData = new FormData();
const inputFilename = "image.png";
const readedFile = fs.readFileSync(inputFilename);
const getStream = (filename) =>{
try{
fs.unlinkSync(filename);
}catch(e){
}
return new WritableStream({
write(chunk) {
return new Promise((resolve, reject) => {
fs.appendFile(filename, chunk, (err) => {
if (err) {
reject(err);
} else {
resolve();
}
});
});
}
});
}
const blobImage = new Blob([readedFile],{
type: "image/png",
});
formData.append('file', blobImage, "image.png");
fetch("https://api.image.antuan01.com/convert",{
method: "post",
body: formData,
}).then( res =>{
const ext = res.headers.get("Content-Type").split('/')[1];
const name= path.parse(inputFilename).name;
res.body.pipeTo(getStream(name + "." + ext));
}).catch(e => console.log(e));